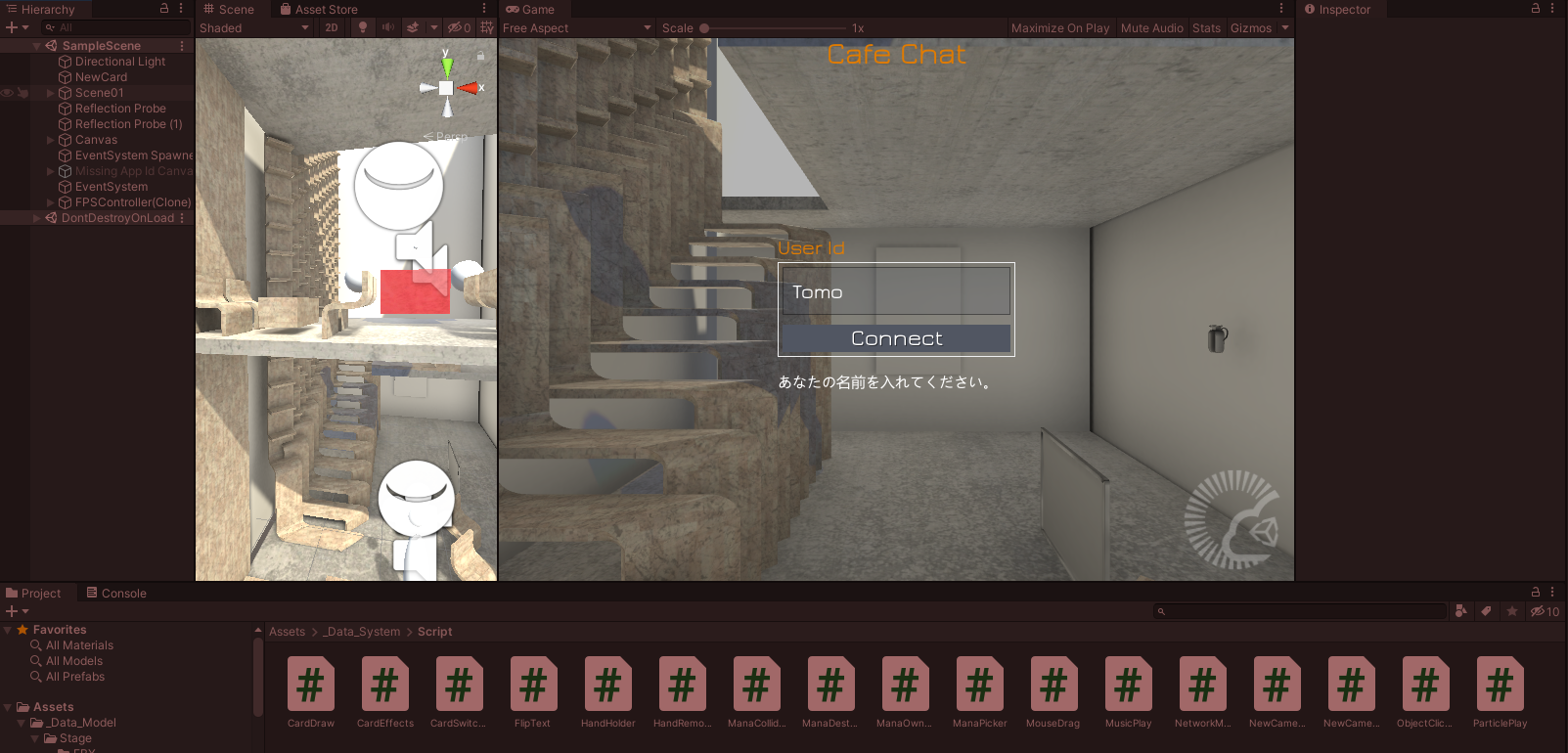
今回は20日間で作った振り返りを行いたいと思います。
書いたC#スクリプトは全部で、16個になりました。
最終的にできた物を全部アップロードしておこうと思います。
今回はスクリプトのみですがご参考まで。
目次
UnityとPUN2のスクリプト一覧
1.CardDraw.cs
using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.EventSystems; using TMPro; using ExitGames.Client.Photon; using Photon.Realtime; public class CardDraw : MonoBehaviourPunCallbacks { public int[] library; [SerializeField] TextMeshPro libraryNum; public GameObject[] myPreMana; public GameObject[] preMana; Vector3 cardSpawnPoint; [SerializeField] Transform libraryPosition; [SerializeField] Transform manaPosition; CardSwitcher cardSwitcher; int playerID; private void Awake() { //現在のポジションをposに格納 Transform pos = transform; //posのpositionをフロートで取得 float x = pos.transform.position.x; float y = pos.transform.position.y; float z = pos.transform.position.z; //取得したフロートに位置をずらす分足す cardSpawnPoint = new Vector3(x, y + 0.02f, z - 0.1f); } private void Start() { libraryNum.text = System.Convert.ToString(library.Length); } public void click() { Draw(); FindPreManaTag(); TransferOwnership(); SendLibraryData(); //RecieveLibraryData(); } public void Draw() { //カードをインスタンス化する GameObject cardCopy = (GameObject)PhotonNetwork.Instantiate("NewCard", cardSpawnPoint, Quaternion.Euler(0, 0, 0), 0); //山札の配列からランダムで一つ要素のインデックスを選ぶ int randomDraw = Random.Range(0, library.Length); //選んだ山札カードのマテリアルをあてがう cardSwitcher = cardCopy.GetComponent<CardSwitcher>(); cardSwitcher.SwitchMaterial(library[randomDraw]); //rigidbody有効化 Rigidbody rigidbody = cardCopy.AddComponent<Rigidbody>(); rigidbody.transform.rotation = Quaternion.Euler(0, 0, 0); rigidbody.constraints = RigidbodyConstraints.FreezeRotation; //山札配列からその要素を一つ削除 var list = new List<int>(); list.AddRange(library); list.Remove(library[randomDraw]); library = list.ToArray(); libraryNum.text = System.Convert.ToString(library.Length); //山札のサイズを変化させる if (library.Length == 0) { Destroy(gameObject); } else { GameObject libraryMesh = GameObject.Find("card"); libraryMesh.transform.localScale = new Vector3(1, library.Length, 1); } } public void FindPreManaTag() { //配列を空にする System.Array.Clear(preMana, 0, preMana.Length); //配列を空にする System.Array.Clear(myPreMana, 0, myPreMana.Length); //"Premana"タグが付いているオブジェクトを配列で全取得 preMana = GameObject.FindGameObjectsWithTag("PreMana"); //配列の回数分下記の処理を繰り返す for (int i = 0; i < preMana.Length; i++) { //i番目の配列のPhotonViewを取得する PhotonView c_PV = preMana[i].GetComponent<PhotonView>(); //i番目の配列のPhotonViewが自分のである場合のみ if (c_PV.IsMine) { //自分のマナトークン配列にゲームオブジェクトを格納する List<GameObject> list = new List<GameObject>(preMana); list.Add(preMana[i]); myPreMana = list.ToArray(); } } } void TransferOwnership() { for (int i = myPreMana.Length-1; i >= 0; i--) { float x = manaPosition.transform.position.x - 0.025f + Random.Range(0, 0.05f); float y = manaPosition.transform.position.y + Random.Range(0, 0.05f); float z = manaPosition.transform.position.z - 0.025f + Random.Range(0, 0.05f); Rigidbody RB = myPreMana[i].GetComponent<Rigidbody>(); RB.constraints = RigidbodyConstraints.None; myPreMana[i].GetComponent<BoxCollider>().enabled = true; myPreMana[i].transform.position = new Vector3(x, y, z); var list = new List<GameObject>(); list.AddRange(myPreMana); list.Remove(myPreMana[i]); myPreMana = list.ToArray(); } } void SendLibraryData() { if (name == "Library1(Clone)") { var hashtable = new ExitGames.Client.Photon.Hashtable(); for (int i = 0; i < library.Length; i++) { hashtable["A" + i] = library[i]; } PhotonNetwork.CurrentRoom.SetCustomProperties(hashtable); } else if(name == "Library2(Clone)") { var hashtable = new ExitGames.Client.Photon.Hashtable(); for (int i = 0; i < library.Length; i++) { hashtable["B" + i] = library[i]; } PhotonNetwork.CurrentRoom.SetCustomProperties(hashtable); } else { Debug.Log("errorLibrary"); } } public override void OnRoomPropertiesUpdate(ExitGames.Client.Photon.Hashtable propertiesThatChanged) { if (name == "Library1(Clone)") { int i = 0; foreach (var prop in propertiesThatChanged) { string key = (string)prop.Key; if (key.Substring(0, 1) == "A") { if (i == 0) { library = new int[0]; } List<int> list = new List<int>(library); list.Add((int)prop.Value); library = list.ToArray(); i++; } } }else if(name == "Library2(Clone)") { int i = 0; foreach (var prop in propertiesThatChanged) { string key = (string)prop.Key; if (key.Substring(0, 1) == "B") { if (i == 0) { library = new int[0]; } List<int> list = new List<int>(library); list.Add((int)prop.Value); library = list.ToArray(); i++; } } } else { Debug.Log("errorCustomProperty"); } if (library.Length == 0) { Destroy(gameObject); } else { GameObject libraryMesh = GameObject.Find("card"); libraryMesh.transform.localScale = new Vector3(1, library.Length, 1); libraryNum.text = System.Convert.ToString(library.Length); } } public void RecieveLibraryData() { library = new int[0]; for (int i = 0; i < 10; i++) { string str = "item" + i; int libraryitem = (int)PhotonNetwork.CurrentRoom.CustomProperties[str]; List<int> list = new List<int>(library); list.Add(libraryitem); library = list.ToArray(); } } }
2.CardEffects.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class CardEffects : MonoBehaviour { public int SwitchManaCost(int cardindex) { if (cardindex == 0) { return 1; }else if(cardindex == 1) { return 2; } else if (cardindex == 2) { return 3; } else { return 4; } } public void CardEffectMethods(int cardindex) { if (cardindex == 0) { Debug.Log("effect01"); } else if (cardindex == 1) { Debug.Log("effect02"); } else if (cardindex == 2) { Debug.Log("effect03"); } else { Debug.Log("error effect"); } } }
3.CardSwitcher.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class CardSwitcher : MonoBehaviourPunCallbacks, IPunObservable { public int cardIndex; public int manaCost; [SerializeField] Material[] CardMat; public void SwitchMaterial(int x ) { cardIndex = x; gameObject.GetComponent<MeshRenderer>().material = CardMat[cardIndex]; CardEffects cardEffects = GetComponent<CardEffects>(); manaCost = cardEffects.SwitchManaCost(cardIndex); } //ここから同期の処理 void IPunObservable.OnPhotonSerializeView(PhotonStream stream, PhotonMessageInfo info) { if (stream.IsWriting) { stream.SendNext(cardIndex); //cardIndexの情報を送る stream.SendNext(manaCost); //shereManaCostの情報を送る } else { cardIndex = (int)stream.ReceiveNext(); //cardIndexの情報を受信 manaCost = (int)stream.ReceiveNext(); //shereManaCostの情報を受信 SwitchMaterial(cardIndex);//materialに反映 } } }
4.FlipText.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class FlipText : MonoBehaviour { private void Update() { Transform TF = Camera.main.GetComponent<Transform>(); transform.LookAt(TF); } }
5.HandHolder.cs
using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; public class HandHolder : MonoBehaviour { //手札を表示する場所の配列 [SerializeField] Transform[] holders; //現在の手札の配列 public int[] hands; //手札のマテリアルの種別 public int handIndex; private void OnCollisionEnter(Collision collision) { //カードtagが付いているオブジェクトがHandHolderに衝突した場合 if (collision.gameObject.tag == "Card") { // GameObject型の配列に、"Hands"タグのついたオブジェクトをすべて格納 GameObject[] allHands = GameObject.FindGameObjectsWithTag("Hands"); //一旦現在のハンドを全部消去 foreach (GameObject hand in allHands) { PhotonNetwork.Destroy(hand); } //衝突したオブジェクトのCardSwitcherを取得して、カードのインデックスを取得 CardSwitcher cardSwitcher = collision.gameObject.GetComponent<CardSwitcher>(); handIndex = cardSwitcher.cardIndex; //手札の配列に取得したインデックスを加える。 List<int> list = new List<int>(hands); list.Add(handIndex); hands = list.ToArray(); //ぶつかったカードを消去 PhotonNetwork.Destroy(collision.gameObject); //現在の配列に基づいて手札を表示 for (int i = 0; i < hands.Length;i++) { //手札のインスタンス化 GameObject cardCopy = (GameObject)PhotonNetwork.Instantiate("NewCard", holders[i].position, holders[i].rotation, 0); //タグを"Hands"に変える cardCopy.tag = "Hands"; //リジッドボディを切る Rigidbody rigidbody = cardCopy.GetComponent<Rigidbody>(); Destroy(rigidbody); //手札のマテリアル表示をインデックス値通りにする cardSwitcher = cardCopy.GetComponent<CardSwitcher>(); cardSwitcher.SwitchMaterial(hands[i]); } } } }
6.HandRemover.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class HandRemover : MonoBehaviour { //自分のマナトークン配列 public GameObject[] myCost; public GameObject[] costs; void OnMouseDown() { //手札の時にしかこの処理を行わない if (tag == "Hands") { //配列を空にする myCost = new GameObject[0]; costs = new GameObject[0]; //"Cost"タグが付いているオブジェクトを配列で全取得 costs = GameObject.FindGameObjectsWithTag("Cost"); int j = 0; Debug.Log(costs.Length); //配列の回数分下記の処理を繰り返す for (int i = 0; i < costs.Length; i++) { //i番目の配列のPhotonViewを取得する PhotonView c_PV = costs[i].GetComponent<PhotonView>(); //i番目の配列のPhotonViewが自分のである場合のみ if (c_PV.IsMine) { //自分のマナトークン配列にゲームオブジェクトを格納する List<GameObject> list = new List<GameObject>(myCost); list.Add(costs[j]); myCost = list.ToArray(); j++; } } CardSwitcher cardSwitcher = GetComponent<CardSwitcher>(); //自分のマナトークン配列が1より長い場合 if (myCost.Length >= cardSwitcher.manaCost) { Debug.Log(myCost.Length + "and" + cardSwitcher.manaCost); //手札を場札とする処理を呼ぶ HandRemove(); CardEffects cardEffects = GetComponent<CardEffects>(); cardEffects.CardEffectMethods(cardSwitcher.cardIndex); //自分のマナトークン配列から一番目の要素を破壊する for(int i = 0; i < cardSwitcher.manaCost; i++) { PhotonNetwork.Destroy(myCost[i]); } } } } void HandRemove() { //HandHolderの取得 GameObject handHolderObject = GameObject.Find("HandHolder"); HandHolder handHolder = handHolderObject.GetComponent<HandHolder>(); //CardSwitcherのIndexの取得 CardSwitcher cardSwitcher = GetComponent<CardSwitcher>(); var removeNum = cardSwitcher.cardIndex; //Indexと同じものをHandHolderの手札配列から一つ削除 var list = new List<int>(); list.AddRange(handHolder.hands); list.Remove(removeNum); handHolder.hands = list.ToArray(); //手札タグをカードタグに付け替える(場に戻すため) tag = "Card"; //rigidbodyを付け、ひっくり返す、 Rigidbody rigidbody = gameObject.AddComponent<Rigidbody>(); rigidbody.transform.rotation = Quaternion.Euler(0, 0, 180); rigidbody.transform.position = new Vector3(5.47f, 4.425f, 11.276f); //回転を拘束 rigidbody.constraints = RigidbodyConstraints.FreezeRotation; } }
7.ManaCollider.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; using Photon.Realtime; public class ManaCollider : MonoBehaviour { // Start is called before the first frame update private void OnCollisionEnter(Collision collision) { Debug.Log("triggerenter"); if(collision.gameObject.tag == "Mana") { Debug.Log("cost"); collision.gameObject.GetComponent<PhotonView>().RequestOwnership(); collision.gameObject.tag = "Cost"; } } }
8.ManaDestroy.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class ManaDestroy : MonoBehaviour { [SerializeField] ParticleSystem particle; [SerializeField] Transform manaSpawnPoint; private void OnDestroy() { Instantiate(particle, transform.position, Quaternion.Euler(-90, 0, 0)); } }
9.ManaOwnership.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; using Photon.Realtime; public class ManaOwnership : MonoBehaviour, IPunOwnershipCallbacks { PhotonView photonView; private void Awake() { photonView = GetComponent<PhotonView>(); } void OnEnable() { PhotonNetwork.AddCallbackTarget(this); } void OnDisable() { PhotonNetwork.RemoveCallbackTarget(this); } void IPunOwnershipCallbacks.OnOwnershipRequest(PhotonView targetView, Player requestingPlayer) { // 自身が所有権を持つインスタンスで所有権のリクエストが行われたら、常に許可して所有権を移譲する if (targetView.IsMine && targetView.ViewID == photonView.ViewID) { bool acceptsRequest = true; if (acceptsRequest) { targetView.TransferOwnership(requestingPlayer); } else { // リクエストを拒否する場合は、何もしない } } } void IPunOwnershipCallbacks.OnOwnershipTransfered(PhotonView targetView, Player previousOwner) { } void IPunOwnershipCallbacks.OnOwnershipTransferFailed(PhotonView targetView, Player senderOfFailedRequest) { } }
10.ManaPicker.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class ManaPicker : MonoBehaviour { [SerializeField] Transform manaSpawnPoint; // Start is called before the first frame update private void OnTriggerExit(Collider other) { Debug.Log("collision"); PhotonView PV = other.GetComponent<PhotonView>(); if (other.gameObject.tag == "Mana" && PV.IsMine) { other.tag = "Cost"; float x = manaSpawnPoint.position.x - 0.025f + Random.Range(0, 0.05f); float y = manaSpawnPoint.position.y + Random.Range(0, 0.05f); float z = manaSpawnPoint.position.z - 0.025f + Random.Range(0, 0.05f); int xx = Random.Range(0, 180); int yy = Random.Range(0, 180); int zz = Random.Range(0, 180); GameObject mana = (GameObject)PhotonNetwork.Instantiate("Mana", new Vector3(x, y, z), Quaternion.Euler(xx, yy, zz), 0); mana.tag = "PreMana"; Rigidbody RB = mana.GetComponent<Rigidbody>(); RB.constraints = RigidbodyConstraints.FreezePosition; mana.GetComponent<BoxCollider>().enabled = false; ManaRefill(); } } void ManaRefill() { float x = transform.position.x - 0.025f + Random.Range(0, 0.05f); float y = transform.position.y + Random.Range(0, 0.05f); float z = transform.position.z - 0.025f + Random.Range(0, 0.05f); int xx = Random.Range(0, 180); int yy = Random.Range(0, 180); int zz = Random.Range(0, 180); GameObject mana = (GameObject)PhotonNetwork.Instantiate("Mana", new Vector3(x, y, z), Quaternion.Euler(xx, yy, zz), 0); } }
11.MouseDrag.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class MouseDrag : MonoBehaviour { public AudioClip audioClip1; public AudioClip audioClip2; AudioSource audioSource; void OnMouseDrag() { if (tag != "Hands") { //cardの位置をワールド座標からスクリーン座標に変換して、objectPointに格納 Vector3 objectPoint = Camera.main.WorldToScreenPoint(transform.position); //cardの現在位置(マウス位置)を、pointScreenに格納 Vector3 pointScreen = new Vector3(Input.mousePosition.x, Input.mousePosition.y, objectPoint.z); //cardの現在位置を、スクリーン座標からワールド座標に変換して、pointWorldに格納 Vector3 pointWorld = Camera.main.ScreenToWorldPoint(pointScreen); //pointWorld.z = transform.position.z; pointWorld.y = 4.4117f; //cardの位置を、pointWorldにする transform.position = pointWorld; } } private void OnMouseDown() { if (tag != "Hands") { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip1; audioSource.Play(); } } private void OnMouseUp() { if (tag != "Hands") { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip1; audioSource.Play(); } else if (tag == "Hands") { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip2; audioSource.Play(); } } }
12.MusicPlay.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class MusicPlay : MonoBehaviourPunCallbacks { public AudioSource audioSource; public AudioClip audioClip1; public AudioClip audioClip2; public AudioClip audioClip3; private void OnMouseDown() { int i = Random.Range(0, 4); var hashtable = new ExitGames.Client.Photon.Hashtable(); hashtable["M" + i] = i; PhotonNetwork.CurrentRoom.SetCustomProperties(hashtable); } public override void OnRoomPropertiesUpdate(ExitGames.Client.Photon.Hashtable propertiesThatChanged) { foreach (var prop in propertiesThatChanged) { string key = (string)prop.Key; if (key.Substring(0, 1) == "M") { if ((int)prop.Value == 1) { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip1; audioSource.Play(); } else if ((int)prop.Value == 2) { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip2; audioSource.Play(); } else if ((int)prop.Value == 3) { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.clip = audioClip3; audioSource.Play(); } else { audioSource = gameObject.GetComponent<AudioSource>(); audioSource.Stop(); } } } } }
13.NetworkManager.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; using Photon.Realtime; public class NetworkManager : MonoBehaviourPunCallbacks { [SerializeField] Transform spawnPoint; private void Start() { // PhotonServerSettingsの設定内容を使ってマスターサーバーへ接続する PhotonNetwork.ConnectUsingSettings(); } // マスターサーバーへの接続が成功した時に呼ばれるコールバック public override void OnConnectedToMaster() { // "Room"という名前のルームに参加する(ルームが存在しなければ作成して参加する) PhotonNetwork.JoinOrCreateRoom("Room", new RoomOptions(), TypedLobby.Default); } // ゲームサーバーへの接続が成功した時に呼ばれるコールバック public override void OnJoinedRoom() { GameObject cardCopy = (GameObject)PhotonNetwork.Instantiate("FPSController", spawnPoint.position, spawnPoint.rotation, 0); } }
14.NewCameraCollision.cs
using Photon.Pun; using System.Collections; using System.Collections.Generic; using UnityEngine; public class NewCameraCollision : MonoBehaviour { [SerializeField] GameObject Camera01; [SerializeField] GameObject Camera02; [SerializeField] GameObject position02; [SerializeField] Transform libraryPosition; [SerializeField] Transform manaPosition; public int playerID; //OnCollisionEnter() PhotonView PV; private void Awake() { PV = GetComponent<PhotonView>(); } private void Start() { if (!PV.IsMine) { Destroy(gameObject); } } private void OnCollisionEnter(Collision collision) { Debug.Log("collision"); PhotonView c_PV = collision.gameObject.GetComponent<PhotonView>(); // playerID = PhotonNetwork.LocalPlayer.ActorNumber; var hashtable = new ExitGames.Client.Photon.Hashtable(); hashtable["Player1"] = playerID; PhotonNetwork.LocalPlayer.SetCustomProperties(hashtable); // if (collision.gameObject.tag == "Player" && c_PV.IsMine) { Destroy(position02); collision.gameObject.SetActive(false); Camera01.SetActive(true); Camera02.SetActive(false); GameObject library = (GameObject)PhotonNetwork.Instantiate("Library1", libraryPosition.position, libraryPosition.rotation, 0); for (int i = 0; i < 7; i++) { float x = manaPosition.position.x - 0.025f + Random.Range(0, 0.05f); float y = manaPosition.position.y + Random.Range(0, 0.05f); float z = manaPosition.position.z - 0.025f + Random.Range(0, 0.05f); int xx = Random.Range(0, 180); int yy = Random.Range(0, 180); int zz = Random.Range(0, 180); GameObject mana = (GameObject)PhotonNetwork.Instantiate("Mana", new Vector3(x, y, z), Quaternion.Euler(xx, yy, zz), 0); } } } }
15.NewCameraCollision2.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; using Photon.Pun; public class NewCameraCollision2 : MonoBehaviour { [SerializeField] GameObject Camera01; [SerializeField] GameObject Camera02; [SerializeField] GameObject position01; [SerializeField] Transform libraryPosition; [SerializeField] Transform manaPosition; PhotonView PV; public int playerID; private void Awake() { PV = GetComponent<PhotonView>(); } private void Start() { if (!PV.IsMine) { Destroy(gameObject); } } private void OnCollisionEnter(Collision collision) { Debug.Log("collision"); PhotonView c_PV = collision.gameObject.GetComponent<PhotonView>(); // playerID = PhotonNetwork.LocalPlayer.ActorNumber; var hashtable = new ExitGames.Client.Photon.Hashtable(); hashtable["Player2"] = playerID; PhotonNetwork.LocalPlayer.SetCustomProperties(hashtable); // if (collision.gameObject.tag == "Player" && c_PV.IsMine) { Destroy(position01); collision.gameObject.SetActive(false); Camera02.SetActive(true); Camera01.SetActive(false); GameObject library = (GameObject)PhotonNetwork.Instantiate("Library2", libraryPosition.position , libraryPosition.rotation, 0); for (int i = 0; i < 7; i++) { float x = manaPosition.position.x - 0.025f + Random.Range(0, 0.05f); float y = manaPosition.position.y + Random.Range(0, 0.05f); float z = manaPosition.position.z - 0.025f + Random.Range(0, 0.05f); int xx = Random.Range(0, 180); int yy = Random.Range(0, 180); int zz = Random.Range(0, 180); GameObject mana = (GameObject)PhotonNetwork.Instantiate("Mana", new Vector3(x, y, z), Quaternion.Euler(xx, yy, zz), 0); /* PhotonView mana_PV = mana.GetComponent<PhotonView>(); if (!mana_PV.IsMine) { mana.GetComponent<BoxCollider>().enabled = false; Rigidbody RB = mana.GetComponent<Rigidbody>(); RB.isKinematic = true; } */ } } } }
16.ParticlePlay.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; public class ParticlePlay : MonoBehaviour { [SerializeField] ParticleSystem particle; void Update() { if (particle.isStopped) //パーティクルが終了したか判別 { Destroy(this.gameObject);//パーティクル用ゲームオブジェクトを削除 } } }
おわりに
これで全部になります。
文字カウントしてみると20000文字+位でそんなにたくさんは書いてないみたいですね!
少しでも何かのご参考なればと思います。
今回の20日間チャレンジではいろいろと学ぶところが多かったように思います。
まだまだ初心者ですが、これからも貪欲に学んでいきたいと思います。
それでは、また!