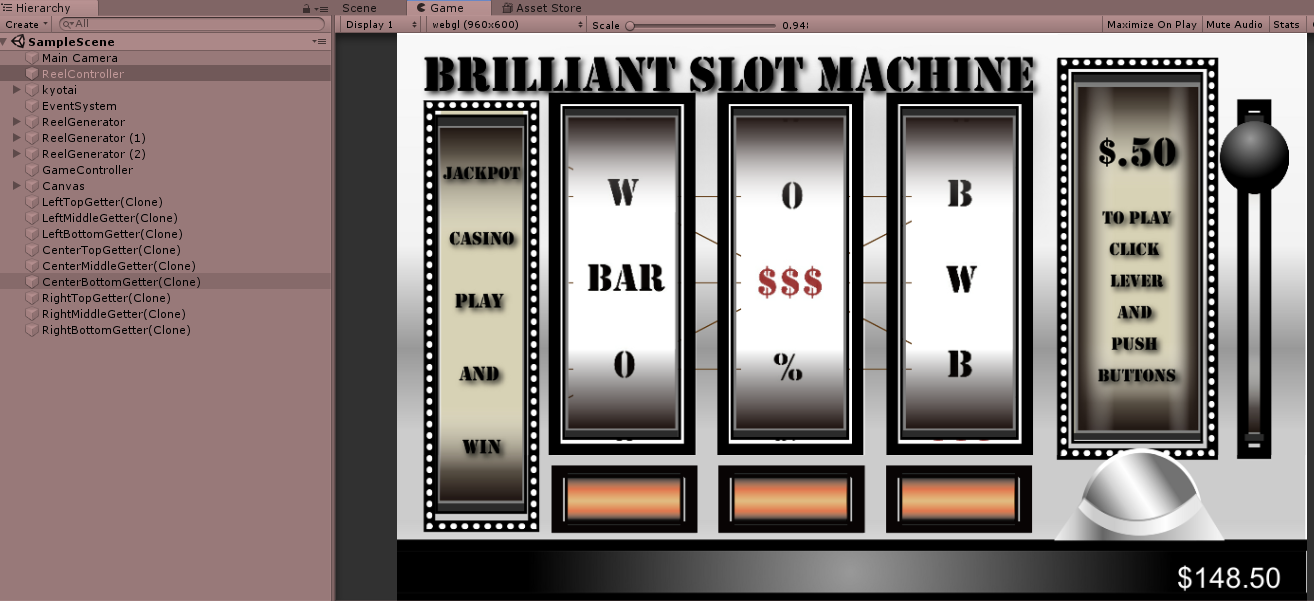
こんにちは、前回はUnityを使った簡単なスロットマシーンの作り方を紹介しました。
今回はその続きの子役の判定とコインのカウント処理などについて考えていこうと思います。
それから子役ができた時には「テッテレー」っていうファンファーレがなったり、リール回転音を追加したりという細かいギミックも加えていきます。
それでは始めましょう!
完成版はこちら↓
目次
スロットマシーンの子役判定の基本的な考え方
子役判定の基本原理
子役を判定するには、例えば中リールの中段に何の柄が止まっているかを判別する必要があります。
これを衝突判定で取得することにします。
上の図のように棒状のBoxCollider2Dを中リール中段に配置します。
しかし、このままでは、常に当たっている絵柄のIDを拾い続けてしまいます。
すると、回っている間に揃ったりしてしまう可能性もありますね。
よって、リールが止まったら棒状のBoxCollider2Dが出てくるようにしたいと思います。
リールを回転させる際にはその都度Destroyするようにします。
スロットマシーンの子役判定の実装準備
子役判定のプレファブ側の準備
まずは衝突判定を実現するために絵柄のプレファブにRigidbody2DとBoxCollider2Dをコンポーネントとして追加します。
BoxCollider2DのIsTriggerにチェックを入れ、Rigidbody2DはGravityScaleを0にして落っこちないようにしておきます。
それから、絵柄IDをプレファブに付けるためにC#スクリプトを付けます。
スクリプトには下記のようにシンプルにpublicの整数を宣言し記載します。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class ReelSymbol : MonoBehaviour { public int symbolNum; }
これによって、インスペクターウィンドウに絵柄IDをタイプすることができます。
この絵柄IDを最終的には約判定プログラムが拾ってくれるということを想定しています。
子役判定の絵柄ID取得プレファブの準備
次に絵柄IDを取得するBoxCollider2dを準備したいと思います。
まず、EmptyObjectを作成し、CenterMiddleGetterと名前を付け、Rigidbody2DとBoxCollider2dをコンポーネントとしてアタッチします。
Edit Colliderボタンを押し、バウンディングボックスを引っ張って棒状にします。
そして、衝突判定を検出するC#スクリプトをアタッチします。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class CenterMiddleGetter : MonoBehaviour { public int centerMiddleSymbol; Reelcontroller reelcontroller;//Reelcontrollerの宣言 private void Awake() { reelcontroller = GameObject.Find("ReelController").GetComponent<Reelcontroller>();//Reelcontrollerの取得 } private void OnTriggerEnter2D(Collider2D other) { if (other.gameObject.CompareTag("reel"))//reelタグが付いたオブジェクトにぶつかったら { ReelSymbol reelSymbol = other.GetComponent<ReelSymbol>(); //ぶつかった相手のReelSymbolにアクセス centerMiddleSymbol = reelSymbol.symbolNum; //symbolNumをcenterMiddleSymbolに代入する Debug.Log("centerMiddle" + centerMiddleSymbol); } } }
Reelタグが付いているGameObjectにぶつかると、絵柄IDを取得し、Public整数のcenterMiddleSymbolに代入するようになっています。
最終的にはこのPublic整数を使って子役の判定を行います。
一度このオブジェクトをシーンビューにドラッグ&ドロップしてインスタンス化し、ヒエラルキービューからプロジェクトウィンドウにもう一度ドラッグ&ドロップしてプレファブ化しておきます。
これによって、後にInstantiateできるようにしています。
絵柄ID取得プレファブのインスタンス化プログラム
この絵柄ID取得コンポーネントをinstantiateするためのプログラムをbuttonを押した時に走らせるようにします。
ボタンを押したタイミングで、そのリールに絵柄ID取得プレファブが出てくるのが理想的です。
それから、ボタンを押した時にポチっと音が鳴るようにしたいと思います。
よってbutton.csを下のように書き換えます。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class button2 : MonoBehaviour { private AudioSource audioSource;//オーディオの宣言 public Reelcontroller reelcontroller; public GameObject centerMiddleGetter;//centerMiddleGetterの宣言 // Start is called before the first frame update void Start() { reelcontroller = GameObject.Find("ReelController").GetComponent<Reelcontroller>(); } public void OnClick() { reelcontroller.stopReel2(); audioSource = gameObject.GetComponent<AudioSource>();//オーディオの取得 audioSource.Play();//ぴこっというオーディオの取得 Instantiate(centerMiddleGetter, new Vector3(5.98f, 1.06f, 0.0f), Quaternion.identity);//中リール中段にインスタンス化 } }
AudioSourceをコンポーネントとして追加し、AudioClipに任意の音ファイルをドラッグ&ドロップします。
音ファイルはフリー素材を使用しています。
最後に、インスペクタービューでbutton2にコンポーネントをドラッグ&ドロップします。
ここまでは中リール中段のみを拾うために書いたプログラムですが、全ての9マスの絵柄を拾うためには中リール中段だけでなく、それぞれ9カ所を拾うように書くことになります。
音の追加と絵柄取得のためのReelControllerへの変更点
取得した絵柄IDをさらに約判定するプログラムGameController(これから作ります)で拾ってあげたいと思います。
拾うタイミングは全てのリールの回転スピードが0である時で、かつ一度だけ行われるようにしたいと思うので、そのようにReelController.csに記載します。
それからリールを回した時に「トゥルルルー」と音が鳴るようにしたいと思います。
3つのリールそれぞれに音を付けたいので、複数の音を使用する場合はAudioSourceコンポーネントを複数追加し、下記のように書くのがセオリ―のようです。
0,1,2はAudioSourceコンポーネントの上から順に0,1,2と認識するようです。
AudioSource[] sounds;//オーディオの宣言 sounds = gameObject.GetComponents<AudioSource>();//オーディオの取得 sounds[0].Play();//リール回転音プレイ sounds[1].Play();//リール回転音プレイ sounds[2].Play();//リール回転音プレイ
上記を踏まえ、以下のようにReelController.csを変更します。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Reelcontroller : MonoBehaviour { AudioSource[] sounds;//オーディオの宣言 public GameObject Reel; //リールを取得 public GameObject Reel2; public GameObject Reel3; Vector3 initialpos; //初期位置 Vector3 initialpos2; Vector3 initialpos3; public float speed1; //リールの回転速度 public float speed2; public float speed3; bool stopflag1 = false;//ボタンが押されたかどうか bool stopflag2 = false; bool stopflag3 = false; bool allflag = true;//子役チェックを一度だけ行うためのbool値 ReelGenerator1 reelGenerator1;//ReelGenerator1の宣言 ReelGenerator1 reelGenerator2; ReelGenerator1 reelGenerator3; GameController gameController; private void Awake() //ゲーム開始時に { sounds = gameObject.GetComponents<AudioSource>();//オーディオの取得 initialpos = this.Reel.transform.position; //初期位置を取得しておく initialpos2 = this.Reel2.transform.position; initialpos3 = this.Reel3.transform.position; reelGenerator1 = GameObject.Find("ReelGenerator").GetComponent<ReelGenerator1>();//ReelGenerator1の取得 reelGenerator2 = GameObject.Find("ReelGenerator (1)").GetComponent<ReelGenerator1>(); reelGenerator3 = GameObject.Find("ReelGenerator (2)").GetComponent<ReelGenerator1>(); gameController = GameObject.Find("GameController").GetComponent<GameController>(); } public void StartReel()//リールを再生成して回し始める関数 { DestroyGetter();//リールを回す時に絵柄ID取得プレファブを破壊する。 reelGenerator1.GenerateReel();//ReelGenerator1のGenerateReel関数を呼ぶ reelGenerator2.GenerateReel(); reelGenerator3.GenerateReel(); speed1 = -0.35f; //リールの回転スピードの代入 speed2 = -0.35f; speed3 = -0.35f; stopflag1 = false; //ボタンを押していない状態にリセット stopflag2 = false; stopflag3 = false; if (stopflag1 != true) { sounds[0].Play();//リール回転音プレイ } if (stopflag2 != true) { sounds[1].Play();//リール回転音プレイ } if (stopflag3 != true) { sounds[2].Play();//リール回転音プレイ } allflag = false; } private void Update() //ゲームが始まったら { this.Reel.transform.Translate(0, speed1, 0); //リールをy方向(下)に動かす this.Reel2.transform.Translate(0, speed2, 0); this.Reel3.transform.Translate(0, speed3, 0); if (Reel.transform.position.y < -130) //リールが一番下に来たら { this.Reel.transform.position = initialpos; //初期位置に戻す } if (Reel2.transform.position.y < -130f) { this.Reel2.transform.position = initialpos2; } if (Reel3.transform.position.y < -130f) { this.Reel3.transform.position = initialpos3; } if (stopflag1 && 0.85 <= Mathf.Abs(Reel.transform.position.y % 1.5f) && Mathf.Abs(Reel.transform.position.y % 1.5f) < 0.88) //ボタンが押されていて、かつ、リールを1.5fで割った余りが規定値以内であったら { speed1 = 0;//リールの回転スピードを0にする } if (stopflag2 && 0.85 <= Mathf.Abs(Reel2.transform.position.y % 1.5f) && Mathf.Abs(Reel2.transform.position.y % 1.5f) < 0.88) { speed2 = 0; } if (stopflag3 && 0.85 <= Mathf.Abs(Reel3.transform.position.y % 1.5f) && Mathf.Abs(Reel3.transform.position.y % 1.5f) < 0.88) { speed3 = 0; } if(speed1 == 0 && speed2 == 0 && speed3 == 0 && allflag != true)//すべてのリールが停止していてallflagがfalseの場合 { allflag = true;//子役チェックが一度しか行われないようにtrueにしておく gameController.CheckMiddle();//子役チェックプログラムの呼び出し } } public void stopReel() //この関数がボタン1を押すと呼ばれる { if (stopflag1 != true)//ボタンがまだ押されてない場合 { speed1 = -0.03f;//リールをゆっくりにする sounds[0].Stop(); } stopflag1 = true; //ボタンを押す } public void stopReel2() { if (stopflag2 != true) { speed2 = -0.03f; sounds[1].Stop(); } stopflag2 = true; } public void stopReel3() { if (stopflag3 != true) { speed3 = -0.03f; sounds[2].Stop(); } stopflag3 = true; } public void DestroyGetter() { //絵柄ID取得プレファブをデストロイしてリセットする関数 GameObject[] getters = GameObject.FindGameObjectsWithTag("getter"); //getterタグが付いているオブジェクトをすべて取得 foreach (GameObject i in getters)//各getterのオブジェクトに対して以下を行う { Destroy(i);//getterの各オブジェクトを破壊する } } }
前回からの変更点を確認します。
まずはStartReel()の中に絵柄ID取得プレファブを破壊するスクリプトの呼び出しを行っています。
それから、フラグをfalseにすることでまだ子役判定がされてない状態にリセットします。
DestroyGetter();//リールを回す時に絵柄ID取得プレファブを破壊する。 allflag = false;
void Update()の中ではリールの回転スピードが全て0でフラグがfalseの場合に、子役チェックプログラムcheckmiddle()の呼び出しが行われるようになっています。
そして、重複したチェックを防ぐためにフラグをtrueにしています。
if(speed1 == 0 && speed2 == 0 && speed3 == 0 && allflag != true)//すべてのリールが停止していてallflagがfalseの場合 { allflag = true;//子役チェックが一度しか行われないようにtrueにしておく gameController.CheckMiddle();//子役チェックプログラムの呼び出し }
最後に絵柄ID取得プレファブをデストロイしてリセットする関数を追加しています。
これが、リールを回転するときに呼ばれることになります。
public void DestroyGetter() { //絵柄ID取得プレファブをデストロイしてリセットする関数 GameObject[] getters = GameObject.FindGameObjectsWithTag("getter"); //getterタグが付いているオブジェクトをすべて取得 foreach (GameObject i in getters)//各getterのオブジェクトに対して以下を行う { Destroy(i);//getterの各オブジェクトを破壊する } }
これでReelController.csへの変更は以上です。
子役判定プログラムGameControllerの作成とコインのカウント
ここでやっと子役判定プログラムCheckMiddle()が出てきます。
ヒエラルキービューで右クリックからEmptyObjectを作成しGameController.csをアタッチします。
全文は後程掲載しますが、要点をかいつまんでご説明します。
LeftTopGetter leftTopGetter; LeftMiddleGetter leftMiddleGetter; LeftBottomGetter leftBottomGetter; CenterTopGetter centerTopGetter; CenterMiddleGetter centerMiddleGetter; CenterBottomGetter centerBottomGetter; RightTopGetter rightTopGetter; RightMiddleGetter rightMiddleGetter; RightBottomGetter rightBottomGetter;
まず、全ての絵柄ID取得プレファブの使用宣言をします。
それから、GetComponentをしておきたいのですが、void Awake()とかStart()時にやってしまうと、まだプレファブができていないのでnullexception Errorが出てしまいます。
よって、プレファブができた後に、GetComponentで取得することにしますので、CheckMiddle()内に以下のように記載します。
leftTopGetter = GameObject.Find("LeftTopGetter(Clone)").GetComponent<LeftTopGetter>();//LeftTopGetterの取得 leftMiddleGetter = GameObject.Find("LeftMiddleGetter(Clone)").GetComponent<LeftMiddleGetter>();//LeftMiddleGetterの取得 leftBottomGetter = GameObject.Find("LeftBottomGetter(Clone)").GetComponent<LeftBottomGetter>();//LeftBottomGetterの取得 centerTopGetter = GameObject.Find("CenterTopGetter(Clone)").GetComponent<CenterTopGetter>();//CenterTopGetterの取得 centerMiddleGetter = GameObject.Find("CenterMiddleGetter(Clone)").GetComponent<CenterMiddleGetter>();//CenterMiddleGetterの取得 centerBottomGetter = GameObject.Find("CenterBottomGetter(Clone)").GetComponent<CenterBottomGetter>();//CenterBottomGetterの取得 rightTopGetter = GameObject.Find("RightTopGetter(Clone)").GetComponent<RightTopGetter>();//RightTopGetterの取得 rightMiddleGetter = GameObject.Find("RightMiddleGetter(Clone)").GetComponent<RightMiddleGetter>();//RightMiddleGetterの取得 rightBottomGetter = GameObject.Find("RightBottomGetter(Clone)").GetComponent<RightBottomGetter>();//RightBottomGetterの取得
クローンとしてできたプレファブを取得するので、文字通り(Clone)といれるのを忘れずに行います。
こんな書き方で任意のタイミングで取得できるのですね。
私はまさかできるとは思っていませんでした。
そして例えば上段がそろった場合を書くのであれば、以下のようにしています。取得したプレファブ内のPublic int値を読みに行って、それが全部一致した場合に、if文が処理されるようになっています。
どの子役かによって番号で分けてあり、それぞれ、音を鳴らし、(時間差で二種類のためCoroutineを使用)それぞれの子役の勝ち関数を呼んであげています。
if (leftTopGetter.leftTopSymbol == centerTopGetter.centerTopSymbol && centerTopGetter.centerTopSymbol == rightTopGetter.rightTopSymbol)//上段の場合 { koyaku = leftTopGetter.leftTopSymbol;//子役のID if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine());//時間差でジリジリという音を入れるコルーチン WinR7();//赤$$$の勝ち関数を呼ぶ } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } }
そして、時間差のコルーチン本体と、それぞれの子役の勝ち関数を下記のように書いています。
ついでに手持ちのコインが増えるように書いています。
予めPublic doubleとして宣言しておく必要はもちろんあります。
IEnumerator SoundCoroutine()//コルーチン本体//普通の子役の場合 { yield return new WaitForSeconds(1.5f); sounds[4].Play(); } IEnumerator Sound7Coroutine()//コルーチン本体//赤$$$と$$$の場合 { yield return new WaitForSeconds(5f); sounds[5].Play(); } } void WinR7()//赤$$$の勝ち関数 { coin += 150;//コインを増やす double win0 = 150.00f;//勝ちテキストを流す勝ちコイン Text win_text = win_object.GetComponent<Text>();//勝ちテキストの取得 win_text.text = "$" + win0.ToString("F2") + "WIN!!";//勝ちテキスト(小数点2桁まで)の内容 } void Win7() { coin += 100; double win1 = 100.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win1.ToString("F2") + "WIN!!"; } void WinBrilliant() { } void WinBell() { coin += 10; double win3 = 10.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win3.ToString("F2") + "WIN!!"; } void WinCherry() { coin += 2.50f; double win4 = 2.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win4.ToString("F2") + "WIN!!"; } void WinWatermelon() { coin += 5; double win5 = 5.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win5.ToString("F2") + "WIN!!"; } void WinReplay() { coin += 1.50f; double win6 = 1.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win6.ToString("F2") + "WIN!!"; }
これで大体要点は網羅していますが、上段、中段、下段、斜め右上、斜め右下の5種類の場合を書かないといけないので、割と膨大な量のスクリプトになります。
説明内容と重複部分がありますが、参考までに一応張り付けておきます。
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class GameController : MonoBehaviour { AudioSource[] sounds;//オーディオの宣言 LeftTopGetter leftTopGetter; LeftMiddleGetter leftMiddleGetter; LeftBottomGetter leftBottomGetter; CenterTopGetter centerTopGetter; CenterMiddleGetter centerMiddleGetter; CenterBottomGetter centerBottomGetter; RightTopGetter rightTopGetter; RightMiddleGetter rightMiddleGetter; RightBottomGetter rightBottomGetter; public double coin; int koyaku; public GameObject score_object; // Textオブジェクト public GameObject win_object; // Textオブジェクト private void Awake() { sounds = gameObject.GetComponents<AudioSource>();//オーディオの取得 } // Update is called once per frame void Update() { Text score_text = score_object.GetComponent<Text>(); score_text.text = "$"+ coin.ToString("F2"); } public void CheckMiddle() { Debug.Log("yobaretayo"); leftTopGetter = GameObject.Find("LeftTopGetter(Clone)").GetComponent<LeftTopGetter>();//LeftTopGetterの取得 leftMiddleGetter = GameObject.Find("LeftMiddleGetter(Clone)").GetComponent<LeftMiddleGetter>();//LeftMiddleGetterの取得 leftBottomGetter = GameObject.Find("LeftBottomGetter(Clone)").GetComponent<LeftBottomGetter>();//LeftBottomGetterの取得 centerTopGetter = GameObject.Find("CenterTopGetter(Clone)").GetComponent<CenterTopGetter>();//CenterTopGetterの取得 centerMiddleGetter = GameObject.Find("CenterMiddleGetter(Clone)").GetComponent<CenterMiddleGetter>();//CenterMiddleGetterの取得 centerBottomGetter = GameObject.Find("CenterBottomGetter(Clone)").GetComponent<CenterBottomGetter>();//CenterBottomGetterの取得 rightTopGetter = GameObject.Find("RightTopGetter(Clone)").GetComponent<RightTopGetter>();//RightTopGetterの取得 rightMiddleGetter = GameObject.Find("RightMiddleGetter(Clone)").GetComponent<RightMiddleGetter>();//RightMiddleGetterの取得 rightBottomGetter = GameObject.Find("RightBottomGetter(Clone)").GetComponent<RightBottomGetter>();//RightBottomGetterの取得 if (leftTopGetter.leftTopSymbol == centerTopGetter.centerTopSymbol && centerTopGetter.centerTopSymbol == rightTopGetter.rightTopSymbol)//上段の場合 { koyaku = leftTopGetter.leftTopSymbol; if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine()); WinR7(); } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } } if (leftMiddleGetter.leftMiddleSymbol == centerMiddleGetter.centerMiddleSymbol && centerMiddleGetter.centerMiddleSymbol == rightMiddleGetter.rightMiddleSymbol)//中段の場合 { koyaku = leftMiddleGetter.leftMiddleSymbol; if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine()); WinR7(); } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } } if (leftBottomGetter.leftBottomSymbol == centerBottomGetter.centerBottomSymbol && centerBottomGetter.centerBottomSymbol == rightBottomGetter.rightBottomSymbol)//下段の場合 { koyaku = leftBottomGetter.leftBottomSymbol; if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine()); WinR7(); } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } } if (leftTopGetter.leftTopSymbol == centerMiddleGetter.centerMiddleSymbol && centerMiddleGetter.centerMiddleSymbol == rightBottomGetter.rightBottomSymbol)//斜め右下の場合 { koyaku = leftTopGetter.leftTopSymbol; if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine()); WinR7(); } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } } if (leftBottomGetter.leftBottomSymbol == centerMiddleGetter.centerMiddleSymbol && centerMiddleGetter.centerMiddleSymbol == rightTopGetter.rightTopSymbol)//斜め右上の場合 { koyaku = leftBottomGetter.leftBottomSymbol; if (koyaku == 0) { sounds[0].Play(); StartCoroutine(Sound7Coroutine()); WinR7(); } else if (koyaku == 1) { sounds[1].Play(); StartCoroutine(Sound7Coroutine()); Win7(); } else if (koyaku == 2) { sounds[2].Play(); WinBrilliant(); } else if (koyaku == 3) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinBell(); } else if (koyaku == 4) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinCherry(); } else if (koyaku == 5) { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinWatermelon(); } else { sounds[3].Play(); StartCoroutine(SoundCoroutine()); WinReplay(); } } IEnumerator SoundCoroutine()//コルーチン本体 { yield return new WaitForSeconds(1.5f); sounds[4].Play(); } IEnumerator Sound7Coroutine()//コルーチン本体 { yield return new WaitForSeconds(5f); sounds[5].Play(); } } void WinR7() { coin += 150; double win0 = 150.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win0.ToString("F2") + "WIN!!"; } void Win7() { coin += 100; double win1 = 100.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win1.ToString("F2") + "WIN!!"; } void WinBrilliant() { } void WinBell() { coin += 10; double win3 = 10.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win3.ToString("F2") + "WIN!!"; } void WinCherry() { coin += 2.50f; double win4 = 2.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win4.ToString("F2") + "WIN!!"; } void WinWatermelon() { coin += 5; double win5 = 5.00f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win5.ToString("F2") + "WIN!!"; } void WinReplay() { coin += 1.50f; double win6 = 1.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = "$" + win6.ToString("F2") + "WIN!!"; } }
最後に、レバーを押した時にコインカウントが1.50$減るようにしておきたいのでlever.csのOnClick()関数の中に下記のように書きました。
gameController.coin -= 1.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = " ";
一応変更点が出たので、lever.csも全文を掲載しておきます。
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; public class Lever : MonoBehaviour { private AudioSource audioSource;//オーディオの宣言 public Reelcontroller reelcontroller;//ReelControllerの宣言 public ReelGenerator1 reelgenerator; //ReelGenerator1の宣言 public GameObject win_object; // Textオブジェクト GameController gameController; void Start() { reelcontroller = GameObject.Find("ReelController").GetComponent<Reelcontroller>();//ReelControllerの取得 reelgenerator = GameObject.Find("ReelGenerator").GetComponent<ReelGenerator1>();//ReelGenerator1の取得 gameController = GameObject.Find("GameController").GetComponent<GameController>();//ReelGenerator1の取得 } public void OnClick() { audioSource = gameObject.GetComponent<AudioSource>();//オーディオの取得 audioSource.Play();//ガチャっというオーディオの取得 this.transform.Translate(0, -4.33f, 0);//レバーを下に落とす StartCoroutine(LeverCoroutine());//コルーチンを始める(レバーを0,5秒後に戻すため) reelgenerator.DestroyReel();//リールを全消しする関数を呼ぶ reelcontroller.StartReel();//リールを再生成して回す関数を呼ぶ gameController.coin -= 1.50f; Text win_text = win_object.GetComponent<Text>(); win_text.text = " "; } IEnumerator LeverCoroutine()//コルーチン本体 { yield return new WaitForSeconds(0.5f);//0.5秒待つ this.transform.Translate(0, 4.33f, 0);//レバーを元に戻す } }
以上で5ラインマシーンの設定終わりです!!
まとめ
いかがでしたでしょうか、少し冗長な書き方になっているかもしれませんが、意外と簡単な理屈ですべてが成り立っている事が分かると思います。
これからは、スロットマシーンの出玉の設定や、確率計算などを行って、台の設定を考えていきたいと思います。
それから、ボーナスステージの表記や、確率変動の場合にプレイヤーに知らせてあげる仕組みなどを盛り込めば、少しは面白いゲームになるかもと思っています。
現時点では、赤$$$が揃う確率は友人の手計算では約1/400以下とかじゃないかという話が出ています。
小さい子役は数回~出るようになっています。
これからもうちょっと面白くなるように絵柄も工夫していきたいと思っています。
ここまで読んで下さりありがとうございます。
今後もご期待ください!